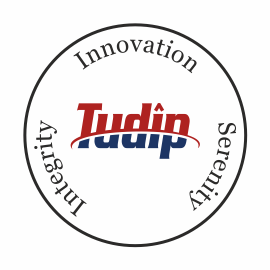
Tudip
24 June 2016
We are putting together few tricks that we routinely use with JQuery Validate. Please note that all the code snippets assumes that the form id is ‘my_form’.
Enable validation on submission:
Call the code snippet below in document.ready.
$("#my_form").validate();
Confirm password validation:
Refer detailed blog ‘JQuery Confirm Password Validation‘ on this topic.
Add STAR to all the required fields:
- You want to display a STAR next to all the fields that are required. Instead of typing in STAR use this code and add it smartly.
- You can also add STAR or something else, by just changing at one place.
- You can also add a class to all the labels indicating the required fields and make them look red using CSS.
Name the text and labels like: <label for="email">E-mail:</label> <input type="text" name="email" id="email" class="textfield required email" /> Then add following code: $(document).ready(function(){ $('.required').each(function(){ id = $(this).attr('id'); $('label[for='+id+']').after('<span>*</span>'); $('label[for='+id+']').addClass('requiredfield-label'); }); });
Cancel form validation on the particular event:
It would disable validations on click on an element with cancel_validation id
$('#cancel_validation').click(function(){ $("#my_form").validate().cancelSubmit = true; });
Do NOT apply form validation on a specific class:
It comes handy especially when you want to disable the validation at run time. This code would suspect validations on all the elements that have ‘ignoreValidation’ class.
$("#my_fomr").validate({ ignore: '.ignoreValidation' });
Customize the placement of the error messages:
In this code, all the error messages will appear inside the element, whose id is error_placeholder.
$("#my_fomr").validate({ errorPlacement: function(error, element) { $("#error_placeholder").append(error); } });
Customized password validation:
Password validation (Minimum 6 character, with at least 1 alphabet and 1 digit)
$.validator.addMethod("my_password_validation", function(value, element) { return this.optional(element) || /^\w*(?=\w*\d)(?=\w*[a-zA-Z])\w*$/.test(value); }, "Password should contain atleast 1 alphabet and 1 digit.");
Now give the class = “my_password_validation” to the password field and set minlength = 6
Override validation messages:
You can override each and every validation message in following way.
jQuery.extend(jQuery.validator.messages, { required: "This field is required.", remote: "Please fix this field.", email: "Please enter a valid email address.", url: "Please enter a valid URL.", date: "Please enter a valid date.", dateISO: "Please enter a valid date (ISO).", number: "Please enter a valid number.", digits: "Please enter only digits.", creditcard: "Please enter a valid credit card number.", equalTo: "Please enter the same value again.", accept: "Please enter a value with a valid extension.", maxlength: jQuery.validator.format("Please enter no more than {0} characters."), minlength: jQuery.validator.format("Please enter at least {0} characters."), rangelength: jQuery.validator.format("Please enter a value between {0} and {1} characters long."), range: jQuery.validator.format("Please enter a value between {0} and {1}."), max: jQuery.validator.format("Please enter a value less than or equal to {0}."), min: jQuery.validator.format("Please enter a value greater than or equal to {0}.") });