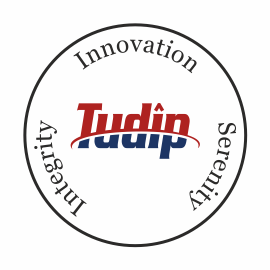
Tudip
24 June 2016
- What are RESTful Web Services?
- When should we use REST?
- When to use SOAP based design?
- JAX-RS (Java API for RESTful Web Services).
- Introducing Jersey.
- Simple Application using Jersey.
What are RESTful Web Services?
REST stands for Representational State Transfer. It’s a key idiom that embraces a stateless client-server architecture in which the web services are viewed as resources and can be identified by their URLs. REST was first described by Roy Fielding in the year 2000.
Wikipedia Says “REST is an architectural style consisting of a coordinated set of constraints applied to components, connectors, and data elements, within a distributed hypermedia system.
REST ignores the details of component implementation and protocol syntax in order to focus on the roles of components, the constraints upon their interaction with other components, and their interpretation of significant data elements.”
HTTP Methods GET, POST, PUT, DELETE are the common HTTP methods that are used with the web services.
When should we use REST?
A RESTful design may be appropriate when
- The web services are completely stateless, which they should be anyway.
- When bandwidth is important and is limited. REST is useful for limited profile devices such as mobiles and PDAs.
- When caching mechanism can be leveraged for enhancing the performance. For example for the responses that are not dynamic can be cached to increase the performance.
When to use SOAP based design?
- SOAP based design is appropriate when a formal contract must be established to describe the interface that the web service offers. The WSDL (Web Service Description Language) describes the details such as messages, operations, bindings, and location of webservice.
- When the architecture must address complex non-functional requirements. For example, Security, transactions etc. With RESTful approach in such scenarios, developers must build this into the application layers themselves.
- When the architecture needs to handle asynchronous processing and invocation. In such cases, the infrastructure provided by the standards such as WSRM and APIs such as JAX-WS with their client-side asynchronous invocation support can be leveraged out of the box.
JAX-RS
The JAX-RS stands for Java API for RESTful Web Services. JAX-RS is a specification that provides support for creating web services based on REST (Representational State Transfer) architectural pattern. JAX-RS uses annotations (introduced in Java SE 5) for the simplification of the development and deployment of web service clients and endpoints.
Introducing Jersey
Developing RESTful Web services that seamlessly support exposing your data in a variety of representation media types and abstract away the low-level details of the client-server communication is not an easy task without a good toolkit. In order to simplify development of RESTful Web services and their clients in Java, a standard and portable JAX-RS API has been designed. Jersey RESTful Web Services framework is open source, production-ready framework for developing RESTful Web Services in Java that provides support for JAX-RS APIs and serves as a JAX-RS (JSR 311 & JSR 339) reference implementation.
The Jersey framework is essentially the JAX-RS Reference Implementation PLUS. Jersey provides its own APIs that in turn extend the JAX-RS toolkit with additional features and utilities to further simplify the RESTful services and expedite the development. Jersey also exposes numerous extension SPIs so that developers may extend and tweak Jersey to best suit their needs.
Goals of Jersey project can be summarized in the following points:
- Track the JAX-RS API and provide regular releases of production quality Reference Implementations that ships with GlassFish;
- Provide APIs to extend Jersey & Build a community of users and developers; and finally Make it easy to build RESTful Web services utilising Java and the Java Virtual Machine.
OK, enough of fluff. Let’s get down to the actual implementation, please!
First download Jersey from https://jersey.java.net/download.html
Next, let’s create a new sample project using Jersey1 and Eclipse.
- Open Eclipse.
- File->New->Dynamic web project.
- After creating project, extract the downloaded Jersey zip file into a folder.
- Copy all the jad files into your web-inf lib folder.
- Now go to web.xml file and add following two lines
<servlet> <servlet-name>servlet-name</servlet-name> <servlet-class>com.sun.jersey.spi.container.servlet.ServletContainer</servlet-class> <init-param> <param-name>com.sun.jersey.config.property.packages</param-name> <param-value>Package-name</param-value> </init-param> </servlet>
-
Now let’s create a simple example page hello.jsp
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>Jersey Example</title> </head> <body> <form action="http://localhost:8090/jerseyExample/api/hello/test" method="POST"id="myForm"> <label>Enter Name : </label><inputtype="text"name="Name"/><input type="submit"value="Submit"> </form> </body> </html>
-
Create form action class Hello.java.
package com.tudip.api; import javax.ws.rs.Consumes; import javax.ws.rs.FormParam; import javax.ws.rs.POST; import javax.ws.rs.Path; import javax.ws.rs.Produces; import javax.ws.rs.core.MediaType; import javax.ws.rs.core.Response; @Path("/hello") public class Hello extends BaseApi { @POST @Path("/hello") @Consumes({ MediaType.APPLICATION_FORM_URLENCODED }) @Produces(MediaType.APPLICATION_JSON) public Response authCheck(@FormParam("Name") String name){ String output = "Hello : " + name; return Response.status(200).entity(output).build(); } }
-
Now right click on the Hello.jsp page from Eclipse and select “Run on Server” and you should see a screen like this.
-
When you add name (let’s say John Doe) and click on Submit button it will print the name that you had entered.